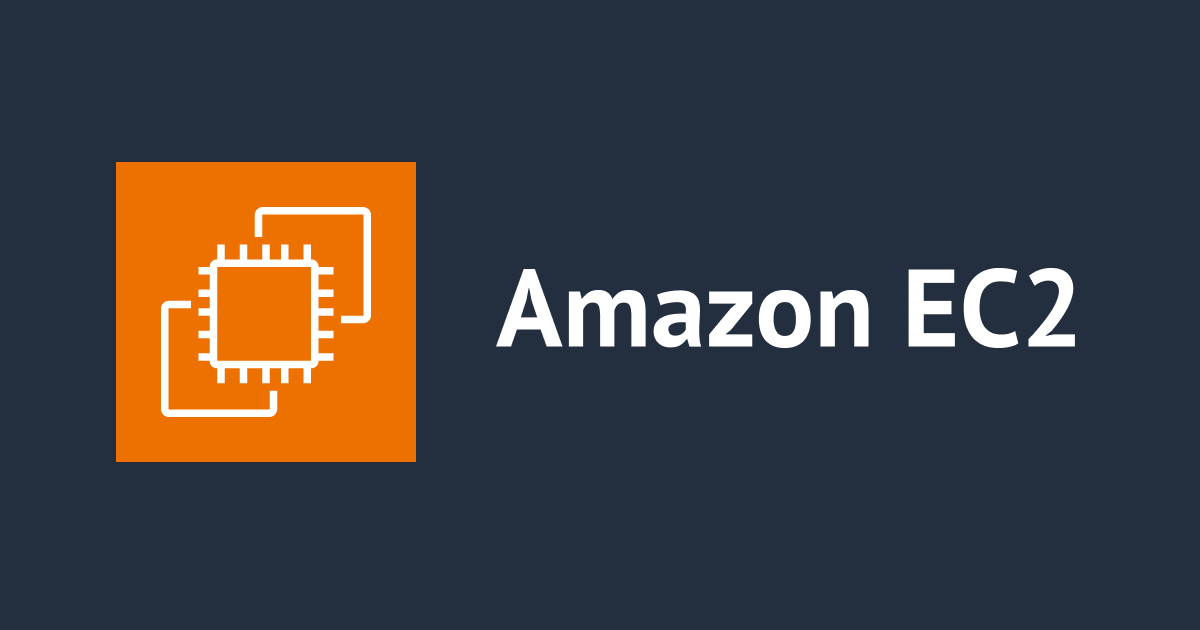
Shell Scriptを使用してAmazon EC2インスタンスをStartとStopする方法。
こんにちは。データアナリティクス事業本部 ビッグデータチームのShiwaniです。
最近、Python スクリプト (boto3)、シェル スクリプトとJava スクリプト を使用して Amazon EC2 インスタンスをStartおよびStopしようとしました。このブログで指定されているタグに基づいて Amazon EC2 インスタンスをStartおよびStopするシェルスクリプト「Shell Script」を共有しています。
前準備
- AWS と通信するために AWS CLI を使います。これらには、セキュリティ認証情報、デフォルトの出力形式、デフォルトの AWS リージョンが含まれます。 次の記事を参照してAWS CLI をインストールしてください。
- インスタンスに安全に接続するためのキーペア「key-pair」を作成します。インスタンスを起動するにはキーペアが必要です。 次の記事を参照してキーペア「key-pair」を作成してください。
Amazon EC2 インスタンスを作成する
マネジメントコンソールからEC2インスタンスを3つ作成します。1つのインスタンスには、「Name=Test」のタグを付け、残りの2つのインスタンスには「Name=Web」のタグを付けます。
Testインスタンスを作成する
「Name=Test」のタグを付けて1つのインスタンスを作成します。
以前に作成されたキーペア「key-pair」を選択します。残りの設定はそのままにしておきます。
Webインスタンスを作成する
「Name=Web」のタグを付けて2つのインスタンスを作成します
以前に作成されたキーペア「key-pair」を選択します。残りの設定はそのままにしておきます。
3つインスタンスが正常に作成されました。
シェルスクリプトを作成する
ファイルを作成する
インスタンスキーペア「key-pair」が保存されているフォルダーに拡張子 .sh のファイルを作成します。
実行する方法
シェルスクリプト「Shell Script」を実行するにはさまざまな方法があります。特定の要件とShell Scriptの機能に基づいて方法を選択してください。
メソッド 1
- スクリプトを実行可能にします。
chmod +x script_name.sh
- スクリプトを実行する。
./script_name.sh
メソッド 2
sh コマンドを使用してスクリプトを実行します。
sh script_name.sh
メソッド 3
bash コマンドを使用してスクリプトを実行する。
bash script_name.sh
スクリプト 1
Amazon EC2 インスタンスをstartする
ここでは、EC2 インスタンスをstartするためのシェルスクリプト「Shell Script」ファイル "ec2_start.sh" を作成しました。 次のスクリプト は「Name=Web」のインスタンスをすべて起動します。
#!/bin/bash TAG_KEY="Name" TAG_VALUE="Web" # Get instance IDs with the specified tag instance_ids=$(aws ec2 describe-instances --filters "Name=tag:${TAG_KEY},Values=${TAG_VALUE}" --query "Reservations[].Instances[].InstanceId" --output text) echo $instance_ids if [ -z "$instance_ids" ]; then echo "No instances found with the tag: ${TAG_KEY}=${TAG_VALUE}" else for instance_id in $instance_ids; do echo "Starting instance with ID: $instance_id" aws ec2 start-instances --instance-ids "$instance_id" done for instance_id in $instance_ids; do # wait for instance to be running echo "Waiting for the instance to start..." aws ec2 wait instance-running --instance-ids "$instance_id" echo "Instance with ID $instance_id has started successfully." done fi
このスクリプトは、AWS CLI を使用して、指定されたタグ (Name=Web) を持つ Amazon EC2 インスタンスを起動します。インスタンス ID を取得し、各インスタンスを起動します。また、スクリプトはインスタンスが実行されるまで待機し、成功メッセージを表示します。
実行
sh コマンドを使用してスクリプトを実行します。
sh ec2_start.sh
結果
i-07a88e1fb77a7d30a i-04203ca4fc602fbd5 Starting instance with ID: i-07a88e1fb77a7d30a { "StartingInstances": [ { "CurrentState": { "Code": 0, "Name": "pending" }, "InstanceId": "i-07a88e1fb77a7d30a", "PreviousState": { "Code": 80, "Name": "stopped" } } ] } Starting instance with ID: i-04203ca4fc602fbd5 { "StartingInstances": [ { "CurrentState": { "Code": 0, "Name": "pending" }, "InstanceId": "i-04203ca4fc602fbd5", "PreviousState": { "Code": 80, "Name": "stopped" } } ] } Waiting for the instance to start... Instance with ID i-07a88e1fb77a7d30a has started successfully. Waiting for the instance to start... Instance with ID i-04203ca4fc602fbd5 has started successfully.
スクリプト 2
Amazon EC2 インスタンスをstopする
ここでは、EC2 インスタンスをstopするためのスクリプトファイル "ec2_stop.sh" を作成します。
#!/bin/bash TAG_KEY="Name" TAG_VALUE="Web" # Get instance IDs with the specified tag instance_ids=$(aws ec2 describe-instances --filters "Name=tag:${TAG_KEY},Values=${TAG_VALUE}" --query "Reservations[].Instances[].InstanceId" --output text) declare -p instance_ids if [ -z "$instance_ids" ]; then echo "No instances found with the tag: ${TAG_KEY}=${TAG_VALUE}" else for instance_id in $instance_ids; do echo "Stopping instance with ID: $instance_id" aws ec2 stop-instances --instance-ids "$instance_id" done for instance_id in $instance_ids; do # wait for instance to be stopped echo "Waiting for the instance to stop..." aws ec2 wait instance-stopped --instance-ids "$instance_id" echo "Instance with ID $instance_id has stopped successfully." done fi
このスクリプトは、AWS CLI を使用して、指定されたタグ (Name=Web) を持つ Amazon EC2 インスタンスを停止します。インスタンス ID を取得し、各インスタンスを停止します。また、スクリプトはインスタンスが停止されるまで待機し、成功メッセージを表示します。
実行
sh コマンドを使用してスクリプトを実行します。
sh ec2_stop.sh
結果
i-07a88e1fb77a7d30a i-04203ca4fc602fbd5 Stopping instance with ID: i-07a88e1fb77a7d30a { "StoppingInstances": [ { "CurrentState": { "Code": 64, "Name": "stopping" }, "InstanceId": "i-07a88e1fb77a7d30a", "PreviousState": { "Code": 16, "Name": "running" } } ] } Stopping instance with ID: i-04203ca4fc602fbd5 { "StoppingInstances": [ { "CurrentState": { "Code": 64, "Name": "stopping" }, "InstanceId": "i-04203ca4fc602fbd5", "PreviousState": { "Code": 16, "Name": "running" } } ] } Waiting for the instance to stop... Instance with ID i-07a88e1fb77a7d30a has stopped successfully. Waiting for the instance to stop... Instance with ID i-04203ca4fc602fbd5 has stopped successfully.
スクリプト 3
Amazon EC2 インスタンスをstart/stopする
ここでは、Amazon EC2 インスタンスをstart/stopするためのshell script ファイル "ec2_start_stop.sh" を作成します。 ユーザー入力に基づいてインスタンスをstartまたはstopできる、より適応性の高いスクリプトが必要な場合は、次のクリプトをお勧めします。
#!/bin/bash TAG_KEY=$1 TAG_VALUE=$2 ACTION=$3 # Check if the action is valid if [ "$ACTION" != "start" ] && [ "$ACTION" != "stop" ]; then echo "Invalid action. Please use 'start' or 'stop'." exit 1 fi # Get instance IDs with the specified tag instance_ids=$(aws ec2 describe-instances --filters "Name=tag:${TAG_KEY},Values=${TAG_VALUE}" --query "Reservations[].Instances[].InstanceId" --output text) if [ -z "$instance_ids" ]; then echo "No instances found with the tag: ${TAG_KEY}=${TAG_VALUE}" else for instance_id in $instance_ids; do echo "${ACTION}ing instance with ID: $instance_id" aws ec2 ${ACTION}-instances --instance-ids "$instance_id" done for instance_id in $instance_ids; do # Wait for the instance status if [ "$ACTION" == "start" ]; then status_command="instance-running" else [ "$ACTION" == "stop" ]; status_command="instance-stopped" fi echo "Waiting for the instance to ${ACTION}..." aws ec2 wait ${status_command} --instance-ids "$instance_id" echo "Instance with ID $instance_id has ${ACTION}ed successfully." done fi
- スクリプトは、TAG_KEY、TAG_VALUE、および ACTION (start または stop) の3つの引数を取ります。
- まずACTIONを検証し、指定されたタグを持つインスタンス ID を取得してから、各インスタンスで指定されたACTION(startまたはstop) を実行します。
- スクリプトはインスタンスが特定のステータス (startの場合は「instance-running」、stopの場合は「instance-stopped」) に達するまで待機し、インスタンスのACTIONごとに成功メッセージを表示します。
- 指定したタグを持つインスタンスが見つからない場合は、適切なメッセージが表示されます。
実行
sh ec2_start_stop.sh Name Test start
結果
starting instance with ID: i-0ab7bf1a15873d56d { "StartingInstances": [ { "CurrentState": { "Code": 0, "Name": "pending" }, "InstanceId": "i-0ab7bf1a15873d56d", "PreviousState": { "Code": 80, "Name": "stopped" } } ] } Waiting for the instance to start... Instance with ID i-0ab7bf1a15873d56d has started successfully.
まとめ
EC2インスタンスを実行/停止するにはさまざまな方法があります。場合によっては、スクリプトを実行して Amazon EC2 インスタンスを起動する必要があり、タスクが完了した後、コストの発生を防ぐためにインスタンスをシャットダウンする必要があります。 このブログで指定されているタグに基づいて Amazon EC2 インスタンスをStartおよびStopする シェルスクリプト「Shell Script」を共有しました。 スクリプト 1 と スクリプト 2 では、簡単にするためにハードコードされたタグ値を使用します。 スクリプト 3 は、ユーザー入力に基づいてインスタンスを開始または停止できる、より汎用性の高いスクリプトを提供します。入力パラメータを調整することで、さまざまなシナリオに使用できます。特定の要件とユースケースに合わせたスクリプトを選択してください。